Availability Per Account Type
Trial
Lite
Pro
White Label
WL – Custom
Introduction
You will be able to use the Schedule_Player() functionto manipulate on what days and time the player will be able to be displayed on any given page within your website.
Notes:
- The dates are inclusive, which means that the video will play on the target dates as well as all of those dates between the targets.
- As with any advanced coding procedures, EZWebPlayer Support staff are available to help our White Label customers.
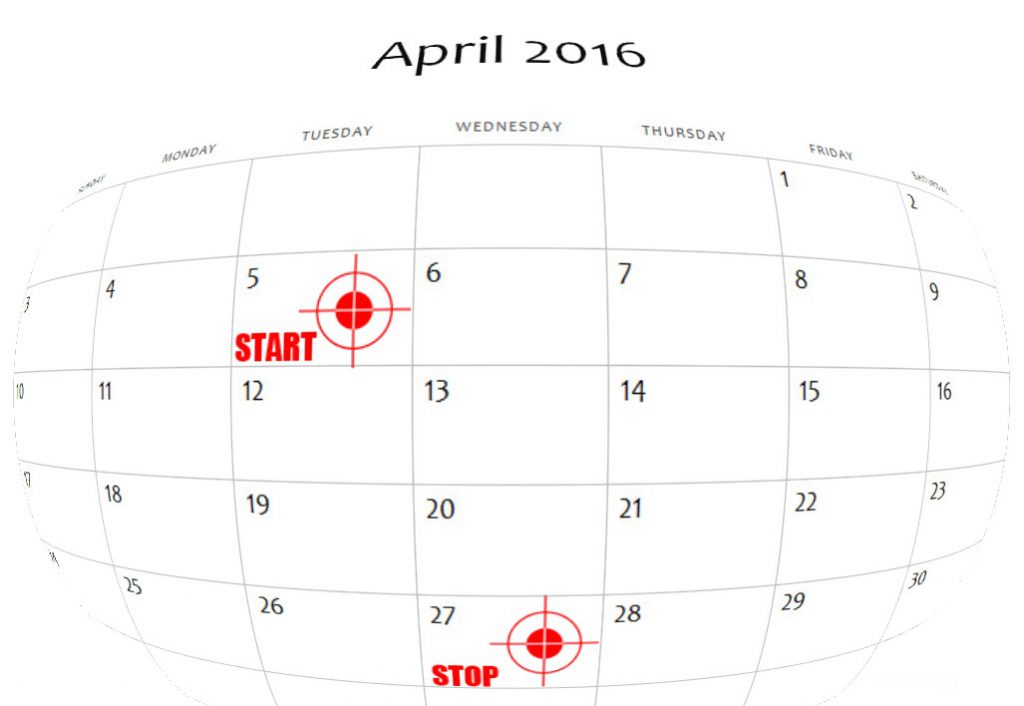
Getting Started
Step 1. Place this script shown below in your website’s header tag.
Sample Code – Click to expand / collapse
<script type="text/javascript">
Schedule_Player = function(PC, CDC, SD, ST, ED, ET){
/*Target the player container and the count down container*/
PC = document.getElementById(PC);
CDC = document.getElementById(CDC);
/*Check if today is between the start date and end date*/
D = new Date();
CD = ((D.getMonth() + 1).toString().length == 1 ? "0" + (D.getMonth() + 1) : (D.getMonth() + 1)) + "/" + (D.getDate().toString().length == 1 ? "0" + D.getDate() : D.getDate()) + "/" + D.getFullYear();
CT = D.getHours() + ":" + D.getMinutes() + ":" + D.getSeconds();
Days = [SD.split("/"), CD.split("/"), ED.split("/")]
Times = [ST.split(":"), CT.split(":"), ET.split(":")]
S = new Date(Days[0][2], Days[0][0]-1, Days[0][1], Times[0][0], Times[0][1], Times[0][2]);
C = new Date(Days[1][2], Days[1][0]-1, Days[1][1], Times[1][0], Times[1][1], Times[1][2]);
E = new Date(Days[2][2], Days[2][0]-1, Days[2][1], Times[2][0], Times[2][1], Times[2][2]);
/*Fill out the cound down container with the time left info*/
CDC.innerHTML = "This video will only be available from " + SD + " " + ST + " to " + ED + " " + ET+".";
/*Display the player or count down timer if today is between the start and end date*/
if(C >= S && C <= E){
PC.style.display = "block";
CDC.style.display = "none";
}else{
PC.style.display = "none";
CDC.style.display = "block";
if(C > E){
clearInterval(Availability_Update);
CDC.innerHTML = "This video is currently not available any more at this time.";
}
}
};
</script>
Step 2. Place this script in the body of your web page where you wish the player to show up.
Sample Code – Click to expand / collapse
<div id="Player" style="Display:none;">
Player Embed Code Here
</div>
<div id="Count_Down"></div>
<script type="text/javascript">
Availability_Update = setInterval( function() {
Schedule_Player("Player", "Count_Down", "00/00/0000", "00:00:00", "00/00/0000", "00:00:00");
}, 1000);
</script>
Step 3. Log into your EZWebPlayer account and grab your players embed code. This will work with the Iframe and Javascript embed codes.
And replace the line from Step 2 that saysPlayer Embed Code Here with your player code.
Sample Code – Click to expand / collapse
<div id="Player" style="Display:none;">
<iframe src="http://ezwp.tv/iframe.htm?v=0000000&w=600&h=450" style="border-width:0;width:600px;height:450px" scrolling="no" allowFullScreen></iframe>
</div>
<div id="Count_Down"></div>
<script type="text/javascript">
Availability_Update = setInterval( function() {
Schedule_Player("Player", "Count_Down", "00/00/0000", "00:00:00", "00/00/0000", "00:00:00");
}, 1000);
</script>
Schedule_Player() – Pass it 5 variables.
Container ID | “Container” | Make sure this matches whatever ID you give to the div surrounding the player embed code. |
---|---|---|
Start Date | “00/00/0000” | Make sure to keep the date in this format.Ex: Jan 2 2016 would be “01/02/2016”. |
Start Time | “00:00:00” | Make sure to keep the time in a 24 hour format.Ex: 1:30AM would be “01:30:00”. |
End Date | “00/00/0000” | Make sure to keep the date in this format.Ex: Dec 31 2016 would be “12/31/2016”. |
End Time | “00:00:00” | Make sure to keep the time in a 24 hour format.Ex: 1:30PM would be “13:30:00”. |